MBScript is the visual scripting system for the game creation tool Modbox. It was designed instead of using a existing text scripting language (like Lua/Javascript) for a few reasons:
- To allow designers to create complex gameplay / AI with drag and drop editing (No syntax errors)
- Can be edited with Keyboard+Mouse, in VR \ AR, with Touch on a mobile device, or with a Gamepad.
- Designed from the start to work online. All code runs online automatically so designers never have to think about networking state
- All changes made to MBScript code are also synced online to other players in real time – and can be edited while playing.
- Unlike node based visual scripting (like Blueprints / Playmaker), MBScript can be switched from visual mode to text mode at any time. A integrated VSCode like text editor (Monaco) is included with Modbox.
- Code can be copied and pasted between the node based Wiring system, which uses MBScript
- It’s event based – with events being any variable changes or ingame events on any entity in the world – so MBScript code can be easily hooked into by other scripts / mods.
- Uses the C# type system to show the correct options and validate the drag and drop blocks
Creating Scripts
- MBScript assets can be created with the Edit Entity Desktop Window or the Edit VR Tool. Each asset creates a new Entity Component type. MBScript Assets can be included in Entity Prefab.
- MBScript can be edited/run at anytime (Running in edit mode can be useful for creating Editor tools / operations. Editing in play mode is a easy way to test changes.)
- MBScript is also used to create Game Managers – which are MBScript assets that are added to the world automatically, and easily accessed by other scripts (like Singletons )
Events
- MBScript code runs on Events, which allows for running code based on any event happening on the Entity or in the world (such as a variable changes value, or a world event happens like a player joins).
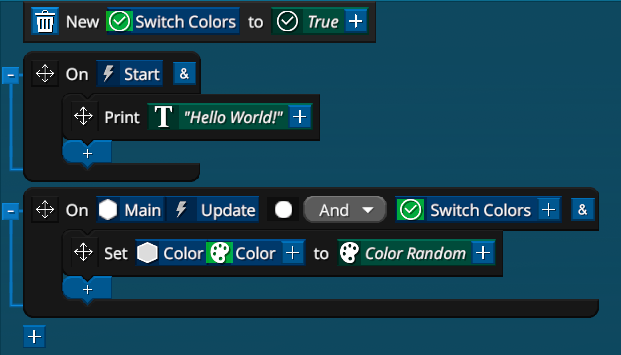
A new variable ‘SwitchColors’ is added, with default value ‘True’. Here the Entity’s Color will be set a random color every Update if ‘SwitchColors’ is True
The ‘On’ lines add Event Lines to run code on events. You can then add other code line types to set values / do operations:
- Set – Set a variable’s value
- Do – Do a method / operation / or event
- If – Run code if a condition is true. Can then add Else lines
- Print – Print a value to the screen, useful for quick testing
- ForEach – Run code on a list. “Do this to everything in this set”. Can run ‘ForEach Entity in the world change it’s material to Wood’
- Wait – Wait a set amount of time before running next code
There are a few other line types: ‘Local’ for temporary variables, Return to stop running code and return from an event, For to do a ‘for’ loop a set number of times, and While to keep looping while a condition is True
Code can run on multiple events and have conditions setting when it runs:
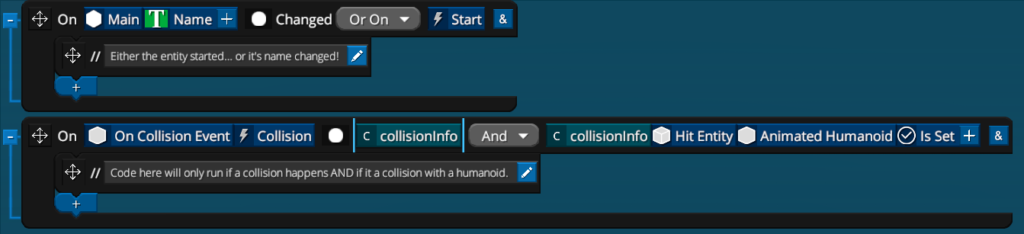
The second event line runs when a Collision happens, and if the collision was with a Animated Humanoid
Selecting Variables
After adding a code line you use the dropdowns to choose a variable or set a value.
After selecting a variable it will show if it still needs the expected type.


The ‘+’ at the end of the line can be used to continue the line with another operation. So because ‘Contains’ returns a True/False, you can then hit the ‘+’ button and select ‘IsFalse’ to invert that return value:

You can keep adding operations like this. Here the ‘Y’ part of the entity’s scale is selected, and set to the same Y value plus a random value:

Variable Options
When selecting a variable in MBScript you select from either:
- Variables created in the Script
- Local variables (added with Local line or as parameters to the Event)
- Components on the Entity and their variables (like it’s Name in the Main component, or Mass in the Physics component)
- Variables in the World (like Gravity, or list of all Material Assets)
- Or Functions – that just return a value. Like ‘Func.Random.Value’ or ‘Func.Mathf.Cos(‘
Tooltips
Hover over a icon to see a description of the variable and it’s value.
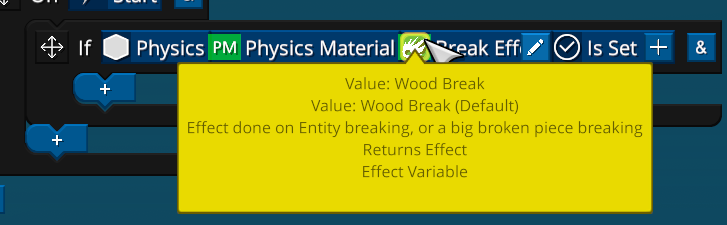
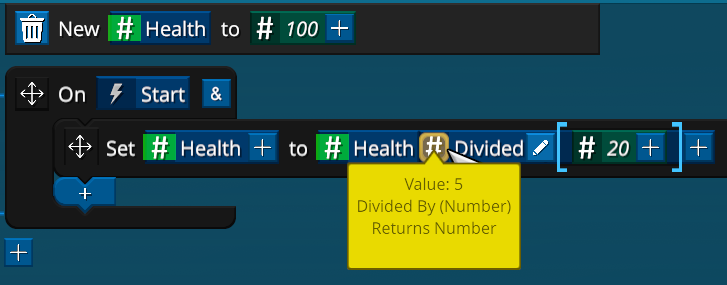
Operations
To change a variable value the Set line can be used to set to a new value (with “Set X to Y”). It can also be useful to do operations directly on the variable:
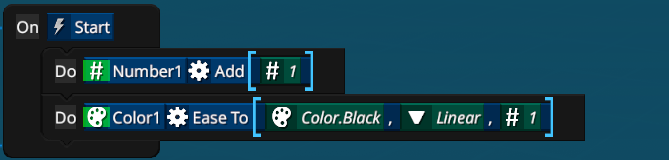
The second line shows using the “Ease To” operation to change a color variable over time. This can be run just once to have it slowly turn color to black.
Lists and Queries
The ‘ForEach’ line can be used to iterate through lists – like the ‘All Entities’ list in the world.
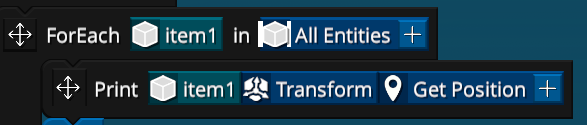


Here it prints the name of all Entities with a mass greater than 1.
Could also keep adding to this, adding a ‘OrderBy’ to order it by mass.
Editing
- Code lines can be dragged by moving the drag handle. Multiple lines can be selected and moved at once. Variables and values can also be dragged to copy them.
- Right click can be used to copy and paste MBScript (which just copies it to the Clipboard as text)
Text Editing
- MBScript can be copy and pasted as text.
- At any time you can switch to text mode to edit, with autocomplete for variable names and immediate error updates.
Here is the same script switched to text mode and edited, checking if the Entity has a ‘Primitive Component’ set on it, and if it does it prints the value of the Entity’s Physics Material Asset :
MBScript Variables
You can add Variables to the MBScript code which will then show in the Entity properties.

To add variables in MBScript, use the ‘+’ button or the ‘Add’ dropdown at the top and select ‘Add Variable’. You then select the variable type.
Variable types that have a value (like Number, Text, ‘Entity’, ‘Entity Asset’) can have a Default Value set (which will be the starting value when the MBScript is added to a Entity).
Variable Types
- Basic types like Bool (True/False), Number, Text
- All Assets types, like Entity Assets, Materials, Audioclips, etc
- Vectors, Range (with a min / max number)
- Entity: for a Entity in the world
Some variable types are made from multiple other types. If ‘Entity Component of X type’ is selected, you then select the type of Entity Component. This could be a component on a Entity like Transform / Health System, or could be another MBScript
Methods and ReturnMethods
Methods can be added that other scripts can call. They can have parameters set to pass values.
ReturnMethod are methods that return a value.
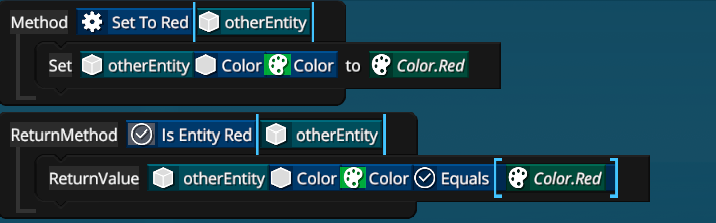
A ‘Is Entity Red’ method returns True if the Entity is red.
Event Variables
Added variables can also include new Events. Events can be called in the same script or in other entities.
Events are used to have any other MBScript code run when it’s called – rather than just specific code like Methods.
If ‘Event(x)’ is selected, then an event variable with a parameter type is added (with the ‘x’ meaning you then select the parameter type). That way the Event can be called passing a value
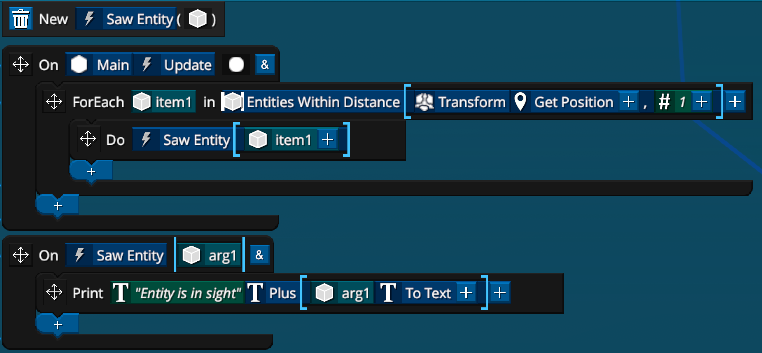
Lists
When creating a variable type with a value there is a option to ‘Create as List’.
If a variable is a List it’ll show borders on the side of the icon.
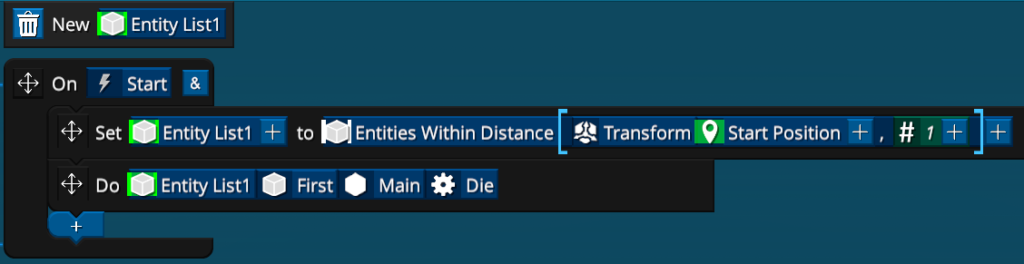
Then the ‘Die’ method is run on the first Entity in the list.
PlayTime Variable
PlayTime variables store the current ‘play mode time’: seconds since play mode started.
Each PlayTime variable also has a event added for when it’s ‘Lapsed’. Which can be a useful way to run code after a set amount of time / on a specific time loop
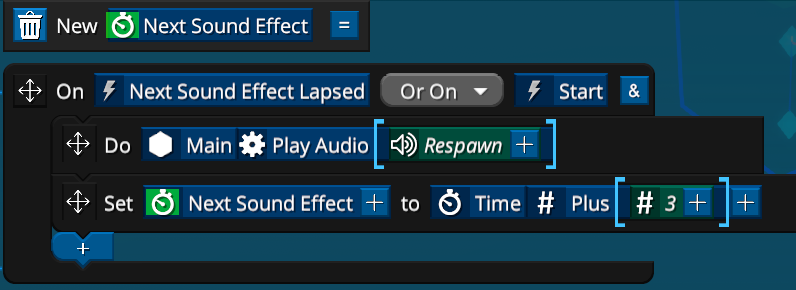
Creating Entities
- Use the Create line to create entities. There are a few Create options like a new Entity from a Asset, or clone a existing entity.
- Create lines have options to set the start position of the entity / rotation. They also have options creating the Entity and staying in it’s ‘Edit Mode’ state, or starting as Disabled
- The Create line creates a new local variable. So you could Create a Entity as disabled or in edit mode, change some of it’s values, then turn Disabled off or turn on it’s play mode.
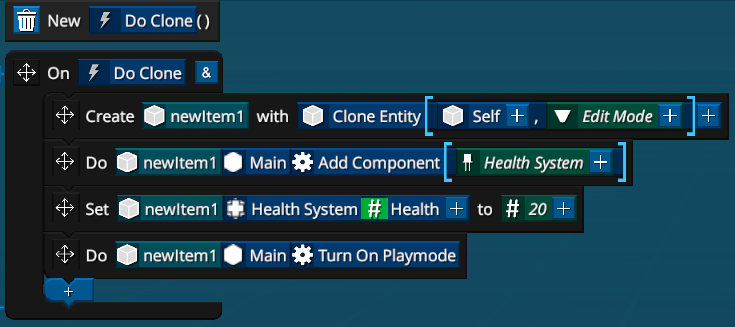
MBScript Attributes
‘Attributes’ can be added to Variables or Event Lines to change some of their settings. Right click or click on the Keyword to then ‘Add Attribute’
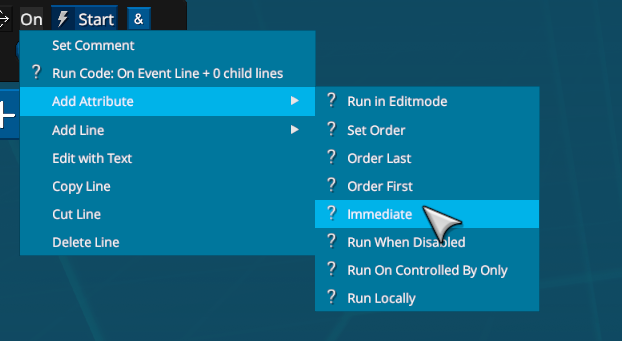
Attributes can be added to Event Lines to set when they run (the order, or if they run locally/online) – hover over the ? to see their description.
By default MBScript code runs on the server – and sends all changed values/methods to all other online players.
This is usually how most code should run – but often you’d want code to run ‘locally’ so it’s more responsive to players (like to play a ‘Damage’ effect immediately on a physics collision, rather than waiting 100ms for server to play Effect).
‘Run On Controlled By’ can be used to have MBScript only run on whatever client is ‘controlling’ the Entity – it’ll then send all changed values to other clients. This can be useful for having responsive feedback to the player’s actions. Players always ‘Control’ their own Avatars and whatever they are holding – then often will Control other entities in the world when they collide with them.
If ‘Run Locally’ is used – the MBScript will run on all clients and no changes will be sent online. This should be used to just show effects / feedback, since it might result in it happening only on some clients (if ‘Run Locally’ is used on a physics collision event it’s possible that only 1 client had that physics collision happen).
Other Attribute options:
- ‘Run In Editmode’ can be used to have event lines run while editing
- Order can be set so that some MBScript Event Lines run before/after others that are linked to the same event.
- ‘Immediate’ will force the MBScript code to run immediately and not a frame later (MBScript code can often be delayed a frame)
Value Attributes
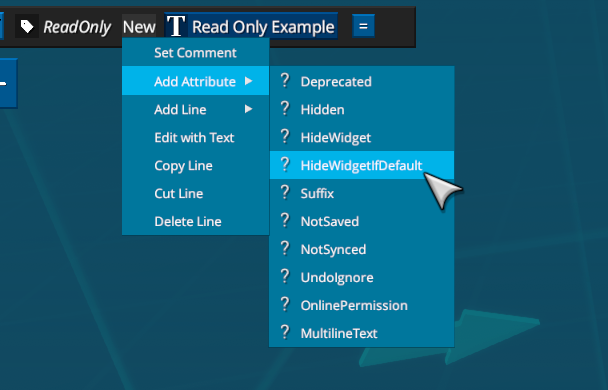
‘ReadOnly’ will set the variable to only be edited inside this script.
Debugging MBScript
Test / Run MBScript
- You can run MBScript while editing it by right clicking and hitting ‘Run Code’
- Using ‘Run Code’ is also a good way to run code manually in edit mode (to do some editing operation)
Breakpoints
‘Debug Mode’ can be used to pause the game when a code line is hit, to allow for seeing values and stepping through code.
Here a ‘Breakpoint’ is added that once hit (on the On Collision’ method), play mode will pause and the scripting window will open to that place in the code.
The icons can be hovered over to see the current value.
“Pause\Breakpoint on Error” in Creation Settings can also be used to pause and highlight and MBScript errors (such as a variable or component not found)
Return Value Events
- Some Components will have a ‘Return Value Event’ so that other components/MBScript can return a Value when a event happens.
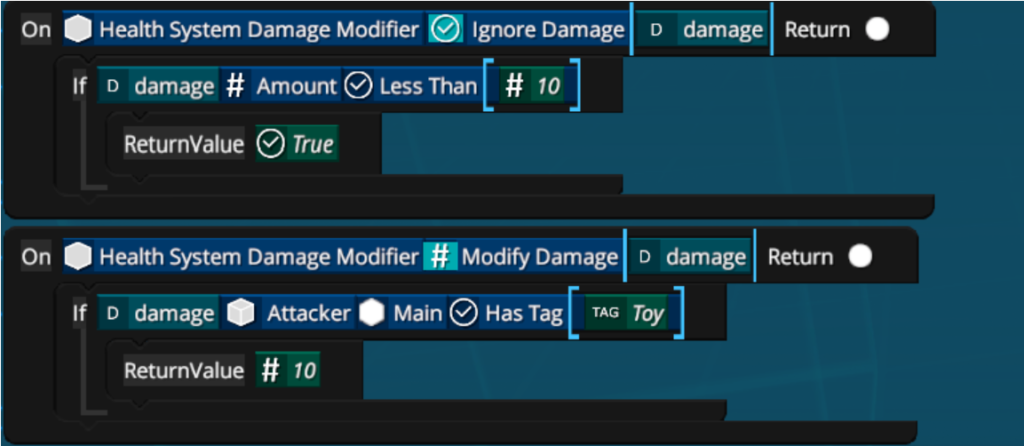
This script can also modify the damage done – setting it to 10 if the damage is from a Toy
- Code running inside a ‘Return Value Event’ can’t change values on the Entity or World, or do operations. The reason for this is so the Return Value can be run at any time (like on hovering over the variable or viewing it in Entity Properties) and not have unexpected side effects.
- ReturnValue Events always run Locally for Players – rather than running just on the server by default like normal MBScript events.
- Multiple Event lines can be added with the same ‘Return Value’ variable. The first ‘ReturnValue’ line will set the value (the order of event lines can be set with MBScript Attributes )
Creating Return Value Event
Use ‘ReturnValue>x’, to create an Event that returns a value. This can also have parameters (if ReturnEvent(y)>x is selected).
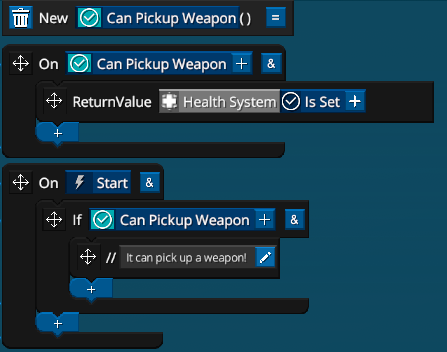
The HealthSystem is shown as greyed out, since this Entity doesnt have it yet.
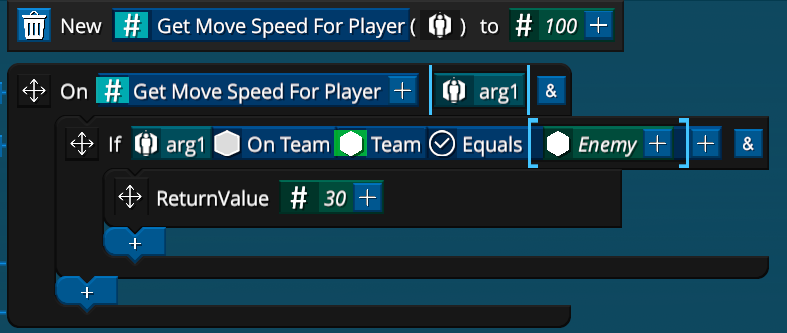
Another script could also then add a Event line to return a value.